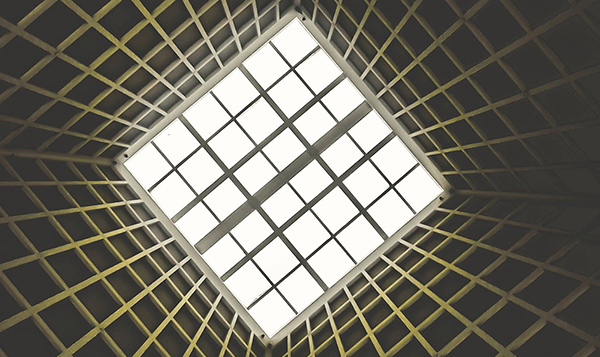
本文是《PyTorch官方教程中文版》系列文章之一,目录链接:[翻译]PyTorch官方教程中文版:目录
本文是对官方英文文档的中文翻译,官方文档链接:TENSORS
张量
张量是一种特殊的数据结构,与数组和矩阵非常相似。在 PyTorch 中,我们使用张量来保存模型的输入和输出以及模型的参数。
张量类似于 NumPy 的 ndarray,并且张量可以在 GPU 或其他硬件加速器上运行。事实上,张量和 NumPy 数组通常可以共享相同的底层内存,从而消除了复制数据的需要(参见 张量和NumPy的互相转换)。张量也针对自动微分进行了优化(我们将在后面的 Autograd 部分看到更多关于这一点的信息)。如果你熟悉ndarrays,那么你就会对Tensor API如指掌。如果没有,请继续阅读本教程。
import torch
import numpy as np
初始化张量
张量可以用多种方式初始化,请查看下面这些例子:
1.用数据直接创建
张量可以直接用数据创建,张量的数据类型将自动推导。
data = [[1, 2],[3, 4]]
x_data = torch.tensor(data)
2.用NumPy数组创建
张量可以用NumPy数组创建,反之亦然(参见 张量和NumPy的互相转换)
np_array = np.array(data)
x_np = torch.from_numpy(np_array)
3.用另一个张量创建
新张量保留参数张量的属性(形状、数据类型),除非显式覆盖它们。
x_ones = torch.ones_like(x_data) # retains the properties of x_data
print(f"Ones Tensor: \n {x_ones} \n")
x_rand = torch.rand_like(x_data, dtype=torch.float) # overrides the datatype of x_data
print(f"Random Tensor: \n {x_rand} \n")
上述代码输出:
Ones Tensor:
tensor([[1, 1],
[1, 1]])
Random Tensor:
tensor([[0.4223, 0.1719],
[0.3184, 0.2631]])
4.用随机值或常量值创建
元组变量“shape”形状是张量的维度,在下面的代码中,它确定输出张量的维数。
shape = (2,3,)
rand_tensor = torch.rand(shape)
ones_tensor = torch.ones(shape)
zeros_tensor = torch.zeros(shape)
print(f"Random Tensor: \n {rand_tensor} \n")
print(f"Ones Tensor: \n {ones_tensor} \n")
print(f"Zeros Tensor: \n {zeros_tensor}")
上述代码输出:
Random Tensor:
tensor([[0.1602, 0.6000, 0.4126],
[0.5558, 0.0912, 0.3004]])
Ones Tensor:
tensor([[1., 1., 1.],
[1., 1., 1.]])
Zeros Tensor:
tensor([[0., 0., 0.],
[0., 0., 0.]])
张量的属性
张量有形状、数据类型和设备属性,设备属性描述了张量所在的设备。
tensor = torch.rand(3,4)
print(f"Shape of tensor: {tensor.shape}")
print(f"Datatype of tensor: {tensor.dtype}")
print(f"Device tensor is stored on: {tensor.device}")
上述代码输出:
Shape of tensor: torch.Size([3, 4])
Datatype of tensor: torch.float32
Device tensor is stored on: cpu
张量的操作
张量有超过100种操作,包括算术、线性代数、矩阵操作(转置、索引、切片)、采样等,详细描述请参考这里。
这些操作中的每一个都可以在 GPU 上运行(速度通常高于 CPU)。如果您使用的是 Colab,请转到运行时>更改运行时类型> GPU 来分配 GPU。
默认情况下,张量是在 CPU 上创建和运行的。我们需要使用 .to 方法显式地将张量转到 GPU执行(在检查 GPU 可用性之后)。请记住,跨设备复制大张量在时间和内存方面的开销都很大。
# We move our tensor to the GPU if available
if torch.cuda.is_available():
tensor = tensor.to('cuda')
请尝试张量的一些操作,如果你熟悉 NumPy API,你会发现 Tensor API 使用起来轻而易举。
类似 numpy 的索引和切片
tensor = torch.ones(4, 4)
print('First row: ',tensor[0])
print('First column: ', tensor[:, 0])
print('Last column:', tensor[..., -1])
tensor[:,1] = 0
print(tensor)
上述代码输出:
First row: tensor([1., 1., 1., 1.])
First column: tensor([1., 1., 1., 1.])
Last column: tensor([1., 1., 1., 1.])
tensor([[1., 0., 1., 1.],
[1., 0., 1., 1.],
[1., 0., 1., 1.],
[1., 0., 1., 1.]])
合并张量
您可以使用“torch.cat”操作沿给定维度合并一系列张量。另请参阅“torch.stack”,这是另一个与“torch.cat”略有不同的张量合并操作。
t1 = torch.cat([tensor, tensor, tensor], dim=1)
print(t1)
上述代码输出:
tensor([[1., 0., 1., 1., 1., 0., 1., 1., 1., 0., 1., 1.],
[1., 0., 1., 1., 1., 0., 1., 1., 1., 0., 1., 1.],
[1., 0., 1., 1., 1., 0., 1., 1., 1., 0., 1., 1.],
[1., 0., 1., 1., 1., 0., 1., 1., 1., 0., 1., 1.]])
算数运算
# This computes the matrix multiplication between two tensors. y1, y2, y3 will have the same value
# 这段代码计算矩阵乘法,y1,y2,y3有相同的值
y1 = tensor @ tensor.T
y2 = tensor.matmul(tensor.T)
y3 = torch.rand_like(tensor)
torch.matmul(tensor, tensor.T, out=y3)
# This computes the element-wise product. z1, z2, z3 will have the same value
# 这段代码逐元素做乘法运算,z1,z2,z3有相同的值
z1 = tensor * tensor
z2 = tensor.mul(tensor)
z3 = torch.rand_like(tensor)
torch.mul(tensor, tensor, out=z3)
单元素张量
如果你有一个单元素张量,例如通过将张量的所有值聚合到一个值中,你可以使用 item() 将其转换为 Python 数值。单元素张量指只有一个元素的张量。
agg = tensor.sum()
agg_item = agg.item()
print(agg_item, type(agg_item))
上述代码输出:
12.0 <class 'float'>
就地操作
将结果存储到操作数中的操作称为就地操作,这些操作都有“_”后缀,例如:x.copy_(y),x.t_(),将更改张量x的值。
print(tensor, "\n")
tensor.add_(5)
print(tensor)
上述代码输出:
tensor([[1., 0., 1., 1.],
[1., 0., 1., 1.],
[1., 0., 1., 1.],
[1., 0., 1., 1.]])
tensor([[6., 5., 6., 6.],
[6., 5., 6., 6.],
[6., 5., 6., 6.],
[6., 5., 6., 6.]])
请注意
就地操作可以节省一些内存,但在计算导数时可能会出现问题,因为会丢失历史记录。因此,不鼓励使用它们。
张量和NumPy的互相转换
CPU上的张量和NumPy数组之间可以共享底层内存,改变其中一个将造成另一个也发生改变。
张量转换为NumPy数组
t = torch.ones(5)
print(f"t: {t}")
n = t.numpy()
print(f"n: {n}")
上述代码输出:
t: tensor([1., 1., 1., 1., 1.])
n: [1. 1. 1. 1. 1.]
改变张量将造成NumPy数组也发生改变:
t.add_(1)
print(f"t: {t}")
print(f"n: {n}")
上述代码输出:
t: tensor([2., 2., 2., 2., 2.])
n: [2. 2. 2. 2. 2.]
NumPy数组转换为张量
n = np.ones(5)
t = torch.from_numpy(n)
改变NumPy数组将造成张量也发生改变:
np.add(n, 1, out=n)
print(f"t: {t}")
print(f"n: {n}")
上述代码输出:
t: tensor([2., 2., 2., 2., 2.], dtype=torch.float64)
n: [2. 2. 2. 2. 2.]
芸芸小站首发,阅读原文:http://www.xiaoyunyun.net/index.php/archives/250.html